java学习笔记(十三) - 常用类
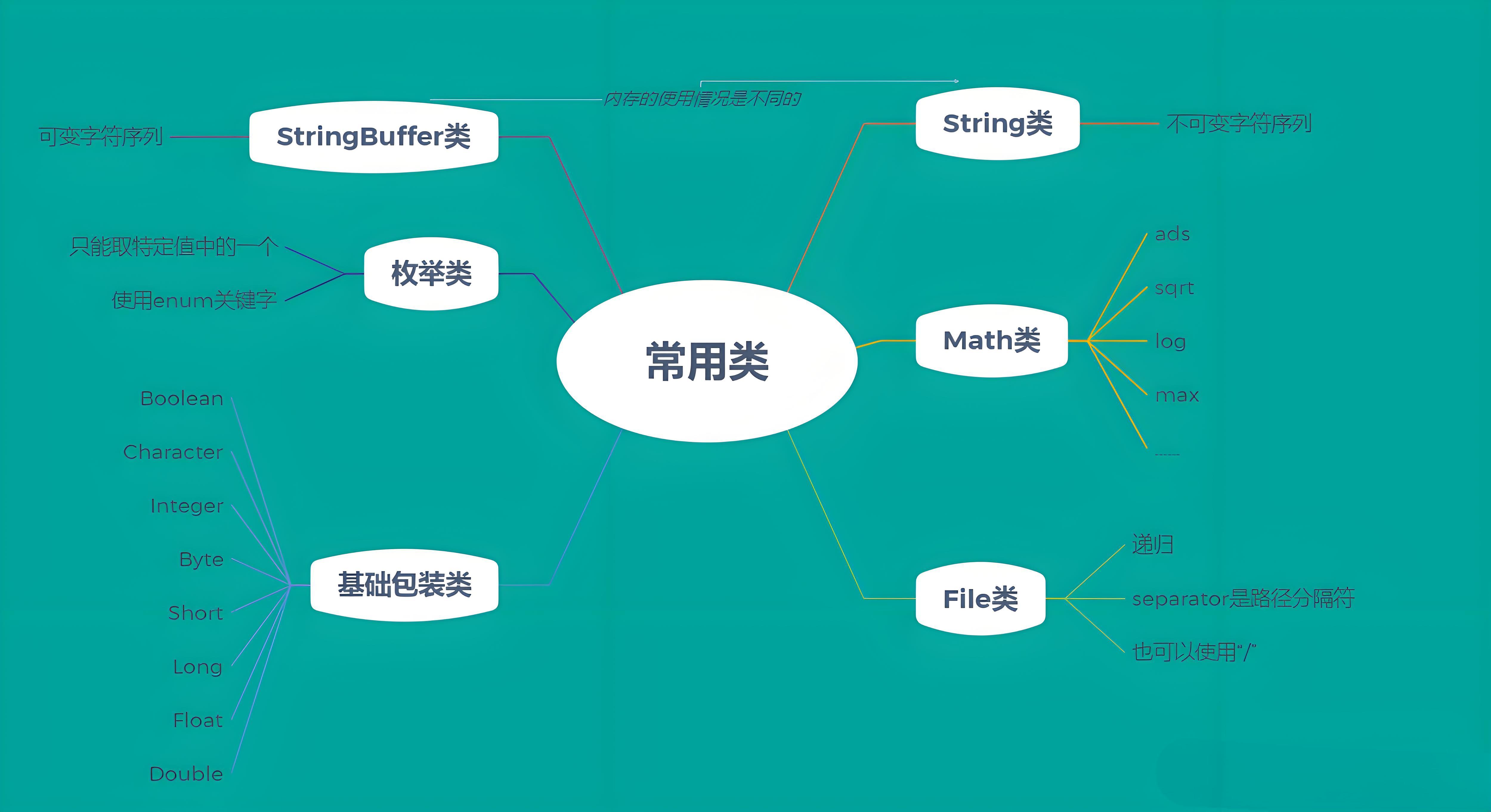
java学习笔记(十三) - 常用类
执笔一、包装类
1. 基本数据类型的包装类
- 八种基本数据类型相应的引用类型–包装类
基本数据类型 | 包装类 |
---|---|
boolean | Boolean |
char | Character |
byte | Byte |
short | Short |
int | Integer |
long | Long |
float | Float |
double | Double |
2. 包装类和基本数据类型转换
2.1 int <----> Integer
2.1.1 手动装箱
-
在jdk5前为手动装箱和手动拆箱 装箱:基本数据类型 --> 包装类型;反之拆箱
1
2
3
4
5
6
7
8//基本数据类型 -----> 包装类型 [手动装箱]
int i = 10;
Integer i1 = new Integer(i);
Integer i2 = Integer.valueOf(i);
//包装类型 ------> 基本数据类型[手动拆箱]
Integer j = new Integer(99);
int j1 = j.intValue();
2.1.2 自动装箱
-
jdk5及以后进行自动装箱和自动拆箱,自动装箱底层调用 valueOf()方法
1
2
3
4
5int n2 = 200;
//自动装箱 int->Integer
Integer integer2 = n2;//底层调用的是 Integer.valueOf(n2);
//自动拆箱 Integer->int
int n3 = integer2;//底层调用 intValue()方法
2.2 String 和 包装类
2.2.1 包装类型 -> String
1 | //包装类型 -> String 类型 |
2.2.2 String - >包装类
1 | //String - >包装类 |
3. Integer类常用方法
1 | System.out.println(Integer.MIN_VALUE);//返回最小值 |
4. Character类常用方法
1 | System.out.println(Character.isDigit('a'));//判断是不是数字 |
二、String类
1. 定义
- String 对象用于保存字符串,也就是一组字符序列
- 字符串常量对象是用双引号括起来的字符序列,如"你好"
- 字符串的字符常用Unicode编码,一个字符(不区分字母还是汉字)占两个字节
- String 类常用的构造方法:
1 | String s1 = new String(); |
- String 是final类 不能被继承
- String 有属性 private final char value[]; 用于存放字符串内容
- value 是一个final类型,不能被修改:即value不能指向新的地址,但是单个字符内容可以变化
1 | String name = "jack"; |
2. 创建String 对象
2.1 直接赋值
1 | String s = "执笔"; |
- 先从常量池查看是否有"执笔" 数据空间,如果有,直接指向;没有就从新创建,然后指向。s最终指向的是常量池的空间地址
2.2 调用构造器
1 | String s2 = new String("执笔"); |
- 先在堆中创建空间,里面维护了value属性,指向常量池的执笔空间,如果常量池没有“执笔”,重新创建;如果有,直接通过value指向。最终指向的是堆中的空间地址
2.3 字符串的特性
-
String 是一个final类,代表不可变的字符序列
-
字符串是不可变的。一个字符串对象一旦被分配,其内容是不可变的
-
intern()方法指向对应字符串的常量池
3. 常用方法
第一组
1 | equals //区分大小写,判断内容是否相等 |
1 | //1. equals 区分大小写,判断内容是否相等 |
第二组
1 | toUpperCase//转换成大写 |
1 | //1. toUpperCase转换成大写 |
三、StringBuffer
1. 介绍
-
java.lang.StringBuffer代表可变的字符序列,可以对字符串内容进行增删
-
很多方法与String相同,但StringBuffer是可变长度的
-
StringBuffer是一个者器
-
StringBuffer是final类
-
实现了Serializable接口,可以保存到文件,或者网络传输
-
继承了抽象类AbstractStringBulider,AbstractStringBulider属性char[] value 存放的字符序列
1 | //1. StringBuffer的直接父类是 AbstractStringBuilder |
2. String 和 StringBuffer对比
-
String保存的是字符串常量,里面的值不能更改,每次String类的更新实际上就是更改地址,效率较低
-
StringBuffer保存的是字符串变量,里面的值可以更改,每次StringBuffer的更新实际上可以更新内容,不用更新地址,效率较高
3. 构造器
1 | //1. 创建一个大小为16的char[] ,用于存放字符内容 |
4. String 和 StringBuffer互相转换
4.1 String --> StringBuffer
1 | //String --> StringBuffer |
4.2 StringBuffer --> String
1 | //StringBuffer ->String |
5. 常用方法
1 | 1) append//增加 |
1 | StringBuffer s = new StringBuffer("hello"); |
四、StringBuilder
1. 介绍
- StringBuilder 继承AbstractStringBuilder 类
- 实现了Serializable,说明StringBuilder对象是可以串行化(对象可以网络传输,可以保存到文件)
- StringBuilder 是final类,不能被继承
- StringBuilder 对象字符序列仍然是存放在其父类AbstractStringBuilder的 char[] vaLue;因此,字符序列是堆中
- StringBuilder 的方法,没有做互斥的处理,即没有synchronized 关键字,因此在单线程的情况下使用
1 | StringBuilder stringBuilder = new StringBuilder(); |
2. String、StringBuffer、StringBuilder比较
- StringBuilder和StringBuffer非常类似,均代表可变的字符序列,而且方法也一样
- String:不可变字符序列,效率低,但是复用率高
- StringBuffer:可变字符序列、效率较高(增删)、线程安全
- StringBuilder:可变字符序列、效率最高、线程不安全
- String使用注意说明:
- string s=“a”; //创建了一个字符串
- s += “b”; //实际上原来的" a"字符串对象已经丢弃了,现在又产生了一个字符串s+ “b” (也就是"ab")。如果多次执行这些改变串内容的操作,会导致大量副本字符串对象存留在内存中,降低效率。如果这样的操作放到循环中,会极大影响程序的性能=>结论:如果我们对String做大量修改,不要使用String
3. String、StringBuffer、StringBuilder的选择
- 如果字符串存在大量的修改操作,一般使用StringBuffer或StringBuilder
- 如果字符串存在大量的修改操作,并在单线程的情况,使用StringBuilder
- 如果字符串存在大量的修改操作,并在多线程的情况,使用StringBuffer
- 如果我们字符串很少修改,被多个对象引用,使用String, 比如配置信息等
五、Math类
- 包含执行基本数学运算的方法
1. 方法
1 | 1) abs//绝对值 |
1 | public class MathMethod { |
六、Arrays类
1. 方法
1 | //(1) toString 返回数组的字符串形式 |
1 | public class ArraysMethod02 { |
2. 排序
1 | public class ArraysSortCustom { |
七、System类
1. 方法
1 | (1) exit//退出当前程序 |
1 | //exit |
八、BigInteger BigDecimal类
- BigInteger适合保存比较大的整型
- BigDecimal适合保存精度更高的浮点型(小数)
1. 方法
1 | add//加 |
1 | BigInteger b1 = new BigInteger(" 1234567890"); |
九、日期类
1. 第一代日期类
- Date:精确到毫秒,代表特定的瞬间
- SimpleDateFormat:格式和解析日期的类,允许进行格式化(日期 - > 文本),解析(文本 - > 日期)和规范化
1 | public class Date01 { |
2. 第二代日期
- 主要是Calendar(日历)
1 | public abstract class Calendar extends Object implements Serializable, |
- Calendar类是一个抽象类,它为特定瞬间与一组诸如YEAR、MONTH、DAY OF MONTH、HOUR等日历字段之间的转换提供了一些方法,并为操作日历字段(例如获得下星期的日期)提供了一些方法。
1 | Calendar C = Calendar.getInstance(); //创建日历类对象//比较简单,自由 |
3. 第三代日期类
1. 方法
1 | (1) LocalDate//获取日期字段 |
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果