java学习笔记 - web基础八(EL表达式)
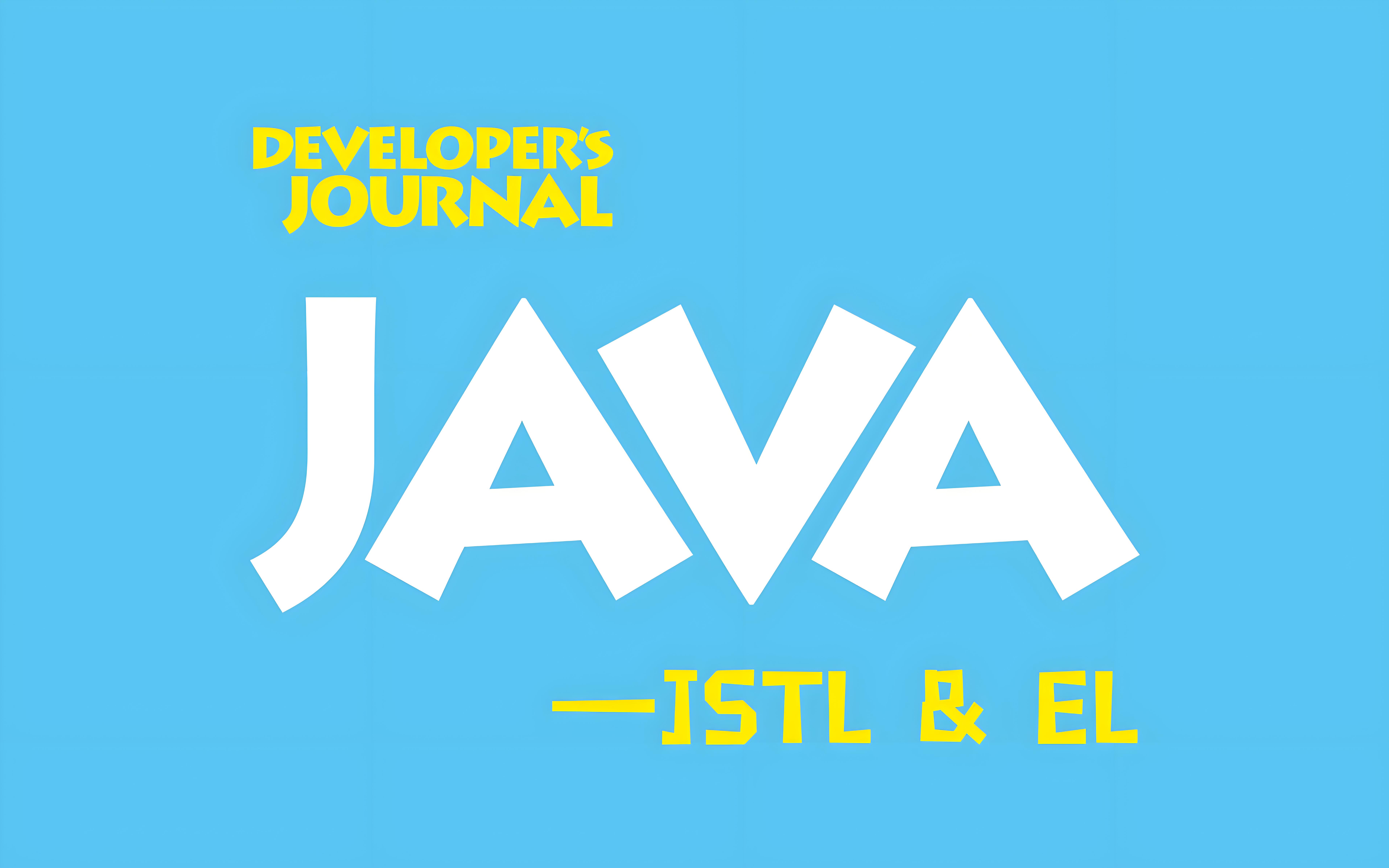
java学习笔记 - web基础八(EL表达式)
执笔EL表达式作用
-
EL 表达式的全称是:Expression Language。是表达式语言
-
EL 表达式的什么作用:EL 表达式主要是代替 jsp 页面中的表达式脚本在 jsp 页面中进行数据的输出
-
EL 表达式的格式是:${表达式}
-
EL 表达式在输出 null 值的时候,输出的是空串。jsp 表达式脚本输出 null 值的时候,输出的是 null 字符串
运算
语法
1 | ${ 运算表达式 } |
关系运算
/关系运算.png)
逻辑运算
/逻辑运算.png)
算术运算
/算术运算.png)
empty运算
- empty 运算可以判断一个数据是否为空,如果为空,则输出 true,不为空输出 false
- 取反为 not empty
1 | <body> |
三元运算
- 表达式 1?表达式 2:表达式 3
- 如果表达式 1 的值为真,返回表达式 2 的值,如果表达式 1 的值为假,返回表达式 3 的值
1 | <!-- 三元运算 --> |
"."点运算和[]中括号运算
- .点运算,可以输出 Bean 对象中某个属性的值
- []中括号运算,可以输出有序集合中某个元素的值
- 并且[]中括号运算,还可以输出 map 集合中 key 里含有特殊字符的 key 的值
1 | <body> |
EL 表达式的 11 个隐含对象
变量 | 类型 | 作用 |
---|---|---|
pageContext | PageContextImpl | 获取 jsp 中的九大内置对象 |
pageScope | Map<String,Object> | 获取 pageContext 域中的数据 |
requestScope | Map<String,Object> | 获取 Request 域中的数据 |
sessionScope | Map<String,Object> | 获取 Session 域中的数据 |
applicationScope | Map<String,Object> | 获取 ServletContext 域中的数据 |
param | Map<String,String> | 获取请求参数的值 |
paramValues | Map<String,String[]> | 获取请求参数的值,获取多个值的时候使用 |
header | Map<String,String> | 获取请求头的信息 |
headerValues | Map<String,String[]> | 获取请求头的信息,它可以获取多个值的情况 |
cookie | Map<String,Cookie> | 获取当前请求的 Cookie 信息 |
initParam | Map<String,String> | 获取在 web.xml 中配置的< context-param >上下文参数 |
四个域
先从最小的作用访问进行选取,作用范围:pageContext
(一个jsp页面)< Request
(一次请求) < Session
(一个会话)< ServletContext
(整个web页面)
- pageScope :pageContext 域
- requestScope :Request 域
- sessionScope :Session 域
- applicationScope :ServletContext 域
pageContext 对象的使用
1 | <body> |
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果