Java学习笔记(十九)- IO流
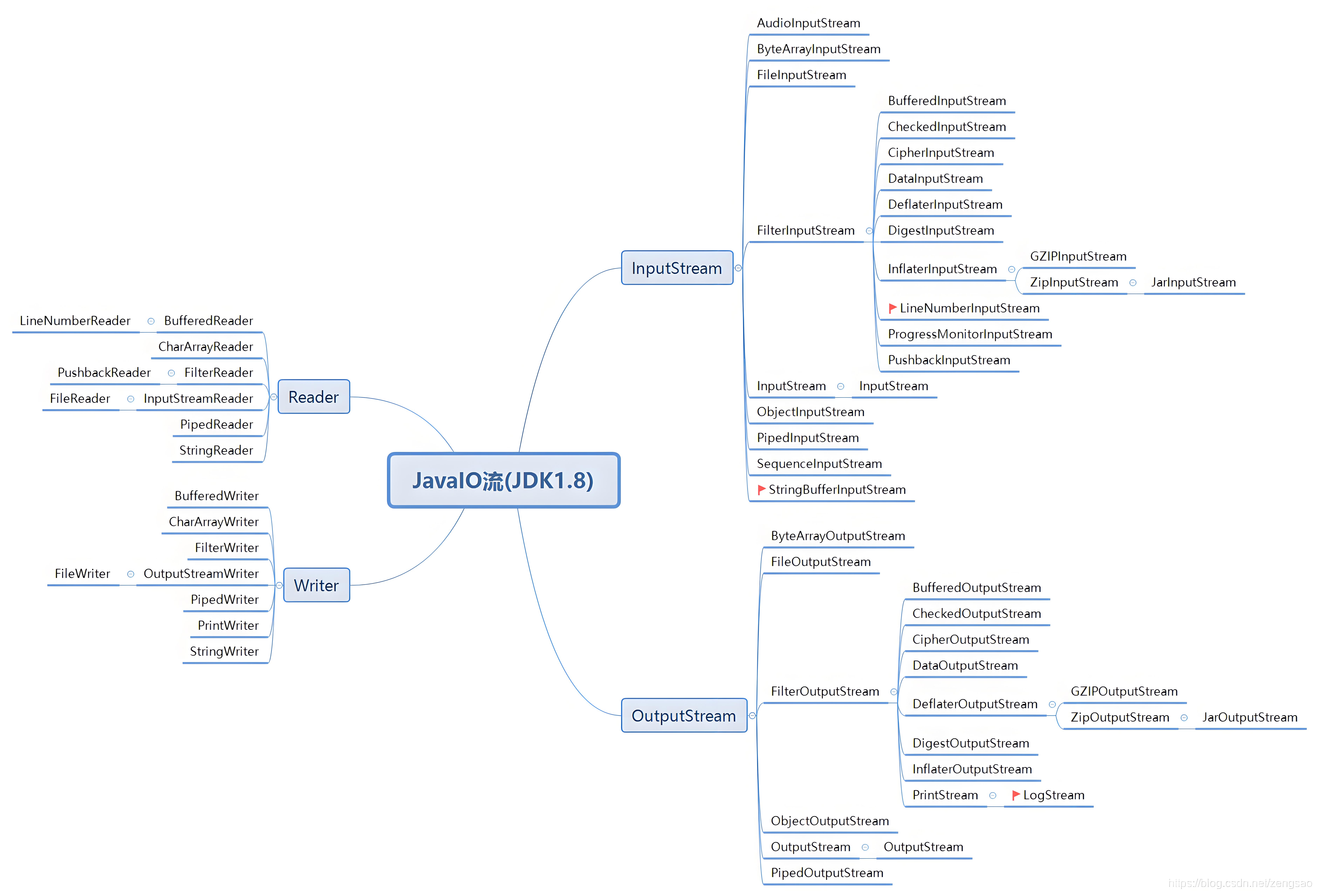
Java学习笔记(十九)- IO流
执笔一、文件
- 保存数据的地方
1. 文件流
-
文件在程序中以流的方式来操作
-
流:数据在数据源(文件)和程序(内存)之间经历的路
- 输入流:数据从数据源(文件)到程序(内存)的路径
- 输出流:数据从程序(内存)到数据源(文件)的路径
-
简化理解
2. 文件操作
2.1 方法
1 | new File(String pathName)//根据路径构建一个File对象 |
1 | //方式一 newFile(String) |
2.2 获取文件信息
1 |
|
二、IO流
1. 原理
- I/O(Input/Output):用于处理数据传输,如读/写文件,网络通讯
- Java程序中,对于数据的输入/输出操作以“流(Stream)”的方式进行
- 在java.io 包下提供了各种“流”类和接口,用于获取不同种类的数据,并通过方法输入输出数据
1.1 IO流体系图
2. 分类
2.1 操作单位
-
字节流(8 bit)二进制文件
-
字符流(按字符)文本文件
2.2 数据流的流向
2.2.1 输入流
(1)InputStream:字节输入流
-
InputStream 抽象类是所有类字节输入流的超类
-
常用子类
-
FileInputStream : 文件输入流
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
public void readFile02() {
String filePath = "j:\\hello.txt";
//字节数组
byte[] buff = new byte[8];//一次读取8个字节
FileInputStream fileInputStream = null;
int readLen = 0;
try {
//创建 FileInputStream 对象 用于读取文件
fileInputStream = new FileInputStream(filePath);
//从该输入流读取b.length字节的数据到字节数组, 如果没有输入可用, 此方法将阻止
//如果返回-1 表示读取完毕
//读取正常 返回实际读取的字节数
while ((readLen = fileInputStream.read(buff)) != -1) {
System.out.print(new String(buff, 0, readLen));//转成char显示
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//关闭文件流, 释放资源
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
} -
BufferedInputStream : 缓冲字节输入流
-
ObjectInputStream : 对象字节输入流
(2)FlieReader : 字符输入流
-
常用方法
1
2
3
4
5
6(1) new FileReader(File/String)
(2) read//每次读取单个字符,返回该字符,如果到文件末尾就返回-1
(3) read(char[])//批量读取多个字符,返回读取到的字符数,如果到文件末尾返回-1
//相关API:
new String(char[])//将char[]转成String
new String(char[],off,len)//将char[]的指定部分转成String-
单字符读取
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
//方式一 单个字符读取
public void fileRead01() {
String filePath = "j:\\story.txt";//文件路径
FileReader fileReader = null;
int data = 0;//用于接收是否读取完成判断
//创建fileRead对象
try {
fileReader = new FileReader(filePath);
//循环读取 每次读取一个字符
while ((data = fileReader.read()) != -1) {
System.out.print((char) data);//将读取到的内容转换成字符输出
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fileReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
} -
字符数组读取
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
//方式二 字符数组读取
public void fileRead02() {
String filePath = "j:\\story.txt";//文件路径
FileReader fileReader = null;
int readLen = 0;//记录读取字符数
char[] buf = new char[8];
//创建FileReader对象
try {
fileReader = new FileReader(filePath);
//循环读取 使用read(buf) 返回的是实际读取到的字符数
//如果返回-1 就说明文件内容读取结束
while ((readLen = fileReader.read(buf)) != -1) {
System.out.print(new String(buf, 0, readLen));//将字符转成字符串输出
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fileReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
-
-
2.2.2 输出流
(1)FileOutputStream : 文件输出流
1 |
|
(2)FileWriter : 字符输出流
-
常用方法
1
2
3
4
5
6
7
8
9(1) new FielWriter(File/String)//覆盖模式,相当于流的指针在首端
(2) new FileWriter(File/string,true)//追加模式,相当于流的指针在尾端
(3) writer(int)//写入单个字符
(4) writer(char[])//写入指定数组
(5) writer(char[],off,len)//写入数组的指定部分
(6) writer(String)//写入字符串
(7) writer(String,off,len)//写入字符串的指定部分
//相关API:String 类
toCharArray//将String 转成char[]- FileWriter 使用后必须要关闭(close)或刷新(flush),否则写入不到指定的文件
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32public class FileWrite_ {
public static void main(String[] args) {
String filePath = "j:\\fileWriter.txt";
//创建FileWriter对象
FileWriter fileWrite = null;
char[] chars = {'1','2','9','8'};
try {
fileWrite = new FileWriter(filePath);//默认写入是覆盖
//(1)writer(int) 写入单个字符
fileWrite.write('H');
//(2)writer(char[]) 写入指定数组
fileWrite.write(chars);
//(3)writer(char[], off, len) 写入数组指定部分
fileWrite.write(chars,0,2);
//(4)writer(string) 写入整个字符串
fileWrite.write("今天天气真好...");
//(5)writer(string, off, len) 写入字符串的指定部分
fileWrite.write("哈喽你好...",0, 2);
System.out.println("写入完成...");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
//fileWriter.flush();
//关闭流 等价于 flush() + close()
fileWrite.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
2.3 流的角色
2.3.1 节点流
- 可以从一个特定的数据源读写数据
- FileReader、FileWriter
2.3.2 处理流(包装流)
(1)功能
- 性能的提高:主要以增加缓冲的方式来提高输入输出的效率。
- 操作的便捷:处理流可能提供了一系列便捷的方法来一次输入输出大批量的数据,使
用更加灵活方便
(2)BufferedReader
(3)BufferedWriter
1 | public class BufferedCopy_ { |
(4)BufferedInputStream
(5)BufferedOutputStream
1 | public class BufferedCopy02 { |
2.3.3 两者关系
- 节点流是底层流/低级流,直接跟数据源相接
- 处理流(包装流)包装节点流,使用了修饰器设计模式,不会直接与数据源相连**[模拟修饰器设计模式]**,既可以消除不同节点流的实现差异,也可以提供更方便的方法来完成输入输出
2.4 对象流
- 用于处理对象
2.4.1 序列化
-
序列化就是在保存数据时,保存数据的值和数据类型
-
需要让某个对象支持序列化机制,则必须让其类是可序列化的,为了让某个类是可序列化的,该类必须实现如下两个接口之一
1
2Serializable //这是一个标记接口,没有方法
Externalizable //该接口有方法需要实现,因此我们一般实现上面的 -
ObjectOutputStream 提供序列化功能
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46//Dog类
//需要可序列化 实现 Serializable接口
public class Dog implements Serializable {
private String name;
private int age;
//序列化对象时,默认将里面所有的属性都进行序列化,但除了static或transient(临时的)修饰的成员
private static String nation;
private transient String color;
//序列化对象时,要求里面的属性的类型也需要实现序列化接口
private Master master = new Master();
//serialVersionUID 序列化的版本号 可以提高兼容性
private static final long serialVersionUID = 1L;
public Dog(String name, int age, String nation, String color) {
this.name = name;
this.age = age;
}
public String toString() {
return "Dog{" +
"name='" + name + '\'' +
", age=" + age +
", color='" + color + '\'' +
'}' + nation;
}
}
//ObjectOutputStream_ 类
public class ObjectOutputStream_ {
public static void main(String[] args) throws Exception {
//.dat 可随意 序列化后 保存的文件格式,不是纯文本 而是它的格式
String filePath = "j:\\data.dat";
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(filePath));
//序列化数据到j:\data.dat
oos.writeInt(100); // int -> Integer (实现了 Serializable) 自动装箱
oos.writeBoolean(true);// boolean -> Boolean (实现了 Serializable)
oos.writeChar('a');// char -> Character (实现了 Serializable)
oos.writeDouble(9.9);// double -> Double (实现了 Serializable)
oos.writeUTF("执笔..."); //String
//保存一个Dog对象
oos.writeObject(new Dog("小黑", 3, "日本", "黑色"));
//关闭流
oos.close();
System.out.println("数据保存完毕(序列化)....");
}
}1
2//seriaVersionUID 序列化的版本号 可以提高兼容性
private static final long serialVersionUID = 1L;
2.4.2 反序列化
-
反序列化就是在恢复数据时,恢复数据的值和数据类型
-
ObjectInputStream 提供反序列化功能
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26public class ObjectInputStream_ {
public static void main(String[] args) throws Exception {
//指定反序列化的文件
String filePath = "j:\\data.dat";
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(filePath));
//读取
//1. 读取(反序列化)的顺序需要和保存的数据(序列化)的顺序一致
//2. 否则会出现异常
System.out.println(ois.readInt());
System.out.println(ois.readBoolean());
System.out.println(ois.readChar());
System.out.println(ois.readDouble());
System.out.println(ois.readUTF());
//dog 的编译类型为 Object 运行类型为 Dog
Object dog = ois.readObject();
System.out.println("运行类型= " + dog.getClass());
System.out.println("dog信息= " + dog);
//细节
//1. 如果我们希望调用Dog的方法,需要向下转型
//2. 需要我们将Dog类的定义,放在到可以引用的位置
Dog dog1 = (Dog) dog;
System.out.println(dog1.getName());
//关闭流
ois.close();
}
}
2.4.3 注意事项
- 读写顺序要一致
- 要求序列化或反序列化对象,需要实现 Serializable
- 序列化的类中建议添加SerialVersionUID,为了提高版本的兼容性
- 列化对象时,默认将里面所有属性都进行序列化,但除了static或transient修饰的成员
- 序列化对象时,要求里面属性的类型也需要实现序列化接口
- 序列化具备可继承性,也就是如果某类已经实现了序列化,则它的所有子类也已经默认实现了序列化
2.5 标准输入输出流
类型 | 默认设备 | |
---|---|---|
System.in 标准输入 | InputStream | 键盘 |
System.out 标准输出 | PrintStream | 显示器 |
1 | public class InputAndOutput { |
2.6 转换流
(1)InputStreamReader
1 | public class InputStreamReader_ { |
(2)OutputStreamWriter
1 | public class OutputStreamWriter_ { |
2.7 打印流
- 打印流只有输出流没有输入流
(1)PrintStream
1 | public class PrintStream_ { |
(2)PrintWriter
1 | public class PrintWriter_ { |
三、Properties类
-
专门用于读写配置文件的集合类
配置文件格式
键=值
键=值
-
键值对不需要有空格,值不需要引号,默认类型是String
1. 常用方法
1 | load//加载配置文件的键值对到Properties对象list:将数据显示到指定设备 |
1.1 读取
1 | public class Properties02 { |
1.2 创建
1 | public class Properties03 { |
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果